Qt Loop Over Widget
I create a program with ten QLabel by Qt Nokia. I want to loop over that QLabel widget and change property over that widget. I can use qFindChild and findChildren function to loop over widget. For example, I have ten QLabel with name label_1, label_2, … , label_10. We can loop over that that widget with code :
for (int i = 1; i <= 10; i++) //loop over ten QLabel { //get QLabel by name QLabel *label = qFindChild<QLabel*>(this, "label_" + QString::number(i)); if (label) //if QLabel exist label->setText("this is label " + QString::number(i)); }
But if we want change property all widget by type (example. loop over QLabel, QLineEdit, etc) we can use function findChildren.
i = 1; //loop over widget by Widget type (ex.QLabel) foreach(QLabel *label, this->findChildren<QLabel*>()) { label->setText("this is label " + QString::number(i)); i = i+1; }
When we create GUI program with Qt Designer (*.ui file) we can use qFindChild and findChildren function to loop over widget. If we create GUI program without Qt Designer (create GUI manually), we can not use qFindChild function to loop over wigdet. We only use findChildren function. If we want to know what name of widget, we can use ObjectName function to get name over widget.
i = 1; //loop over widget by Widget type (ex.QLabel) foreach(QLabel *label, this->findChildren<QLabel*>()) { label->setText("this is label " + QString::number(i)); //this is name of QLabel selected QString nameLabel = label->ObjectName(); i = i+1; }Source : http://www.qtcentre.org/threads/38305-for-loop-application
Related Posts
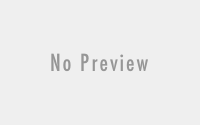
C/C++ : fftw Tutorial
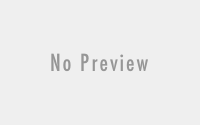
Qt : QProcess get PID
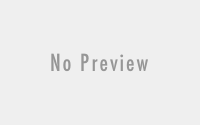