C/C++ Read Binary File
C/C++ Read Binary File
A binary file is a computer file which may contain any type of data, encoded in binary form for computer storage and processing purposes (wikipedia.com). On this article, I will write about C/C++ Read Binary File. We can read binary file and write binary file with any of other data type (read and write binary file as character, read and write binary file as integer, read and write binary file as float, read and write binary file as struct like complex number, etc).
I will create a simple C/C++ read binary file and C/C++ write binary file program. This is a function how C/C++ write binary file float from data float.
/* write data as float binary file */ void writeFloat2BinaryFile(char *filename, float *data, int ndata) { FILE *outfile=NULL; /*open file */ outfile = fopen(filename, "w"); if(!outfile) /*show message and exit if error opening file */ { printf("error opening file %s \n", filename); exit(0); } /*write float array as float binary file */ fwrite(data, sizeof(float), ndata, outfile); /*close file */ fclose(outfile); }
This function C/C++ write binary file show to us how to write a float data (data variable) and save that file to binary float file. If we want to load file from C/C++ write binary file above code, we can use this C/C++ read binary file. This C/C++ read binary will will load a binary file and return float number. Please check this C/C++ read binary file source code :
/* read binary float file, return as array of float */ float *readFloatBinaryFile(char *filename, int ndata) { FILE *infile=NULL; float *dataread; int idx; /*open file */ infile = fopen(filename, "r"); if(!infile) /*show message and exit if fail opening file */ { printf("error opening file %s \n", filename); exit(0); } /*allocated memory/array for float data */ dataread = (float*) calloc(ndata, sizeof(float)); /*read file and save as float data */ fread(dataread, sizeof(float), ndata, infile); /* close file */ fclose(infile); return(dataread); }
This is output from my C/C++ read binary file and C/C++ write binary file with length of data is 5.
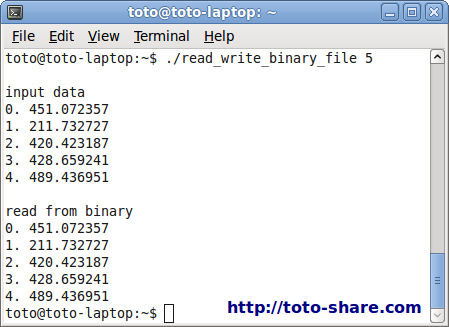
This program will save float data to ascii and binary file. If we check size of this Ascii and binary file, we get this different size of this two file. This is a size from this two file :
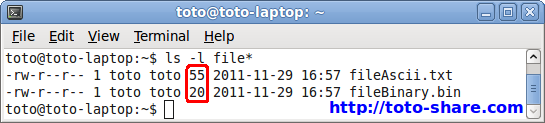
This is explaining from this size of file. Input length of data is 5 data. float number have size 4 byte every data. So, size of binary file is 5×4 byte = 20 bytes.
From above code, the float number have 10 number every data (ex. 451.072357). So, the size of ascii file from this data is 5x10byte=50bytes. Every number have “enter” or newline character. So, size of ascii file is : 50bytes + 5bytes = 55 bytes.
This is a complete C/C++ read binary file and C/C++ write binary file source code above, you can download this code at here.