Android Sent Text To JavaScript
While developing Android application, sometimes we need to use webview and want our android (java code) communicate with html in webview. We can use javascript to communicate between java to html and vice versa. Dont forget to setting javascript enabled in our webview to true.
Please check code below to check how Android sent text to javascript :
public class MainActivity extends AppCompatActivity { public class MyJavaScriptInterface { Context mContext; MyJavaScriptInterface(Context c) { mContext = c; } public void showToast(String toast){ Toast.makeText(mContext, toast, Toast.LENGTH_SHORT).show(); } public void openAndroidDialog(String msg){ AlertDialog.Builder myDialog = new AlertDialog.Builder(MainActivity.this); myDialog.setTitle("Text From JavaScript"); myDialog.setMessage(msg); myDialog.setPositiveButton("OK", null); myDialog.show(); } } WebView webView; EditText editText; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //webview initialization webView = (WebView)findViewById(R.id.webView); final MyJavaScriptInterface myJavaScriptInterface = new MyJavaScriptInterface(this); webView.addJavascriptInterface(myJavaScriptInterface, "AndroidFunction"); webView.getSettings().setJavaScriptEnabled(true); webView.loadUrl("file:///android_asset/index.html"); //sent data from android to javascript editText = (EditText)findViewById(R.id.editText); Button button = (Button)findViewById(R.id.button); button.setOnClickListener(new Button.OnClickListener() { @Override public void onClick(View arg0) { // TODO Auto-generated method stub String msgToSend = editText.getText().toString(); webView.loadUrl("javascript:callFromActivity(\"" + msgToSend + "\")"); } }); } }
Create file index.html code in assets directory. This is the contents from this html Android sent text to javascript file
<html> <head> <script type="text/javascript" src="myscript.js"></script> </head> <body style="background-color:powderblue;"> Text From Android : <br> <input id="txtBoxImport" readonly> <br> <br> Sent Text To Android : <br> <input id="txtBoxExport"> <br> <br> <input name="btnSent" type="button" value="Sent" onClick="sent_to_android()"> </body> </html>
Dont forget to create javascript file with name myscript.js and save to assets directory. This javascript is the important part, becuase used to communicate between java android and javascript (webview).
function sent_to_android() { msg = document.getElementById("txtBoxExport").value; AndroidFunction.openAndroidDialog(msg); } function callFromActivity(msg){ document.getElementById("txtBoxImport").value = msg; }
Please check below image for output Android sent text to javascript code :
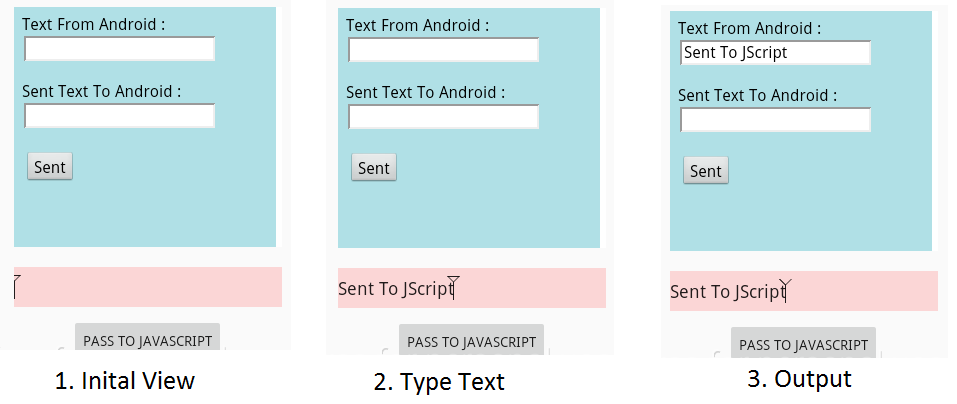
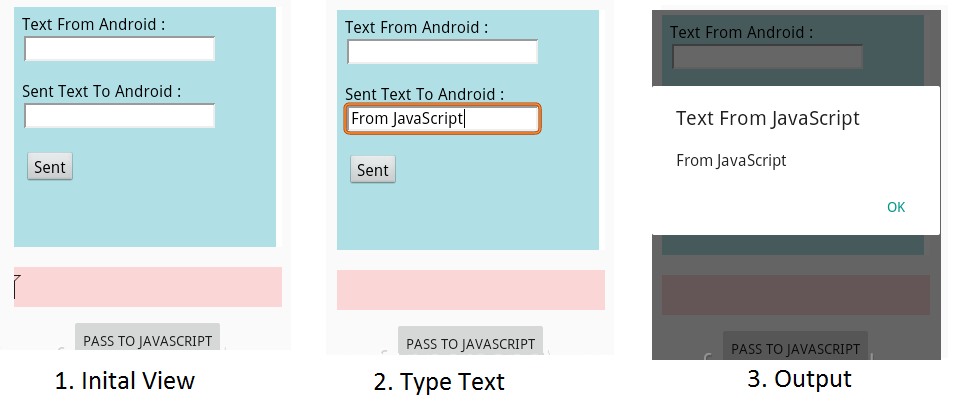
This is a simple tutorial how Android sent text to javascript and how to javascript or webview sent text to Android. I hope this tutorial can help you solve your problem. You can download the compete code Android sent text to javascript in here.
Source : http://www.cuelogic.com/blog/simple-android-java-javascript-bridge/
Hi
Great post! I learned something new and interesting, which I also happen to cover on my blog. It would be great to get some feedback from those who share the same interest about Airport Transfer, here is my website ZQ3 Thank you!
is this right. my has been erased by the looks of itcan you see the problem